Effective Data Collection Methods for Data Science
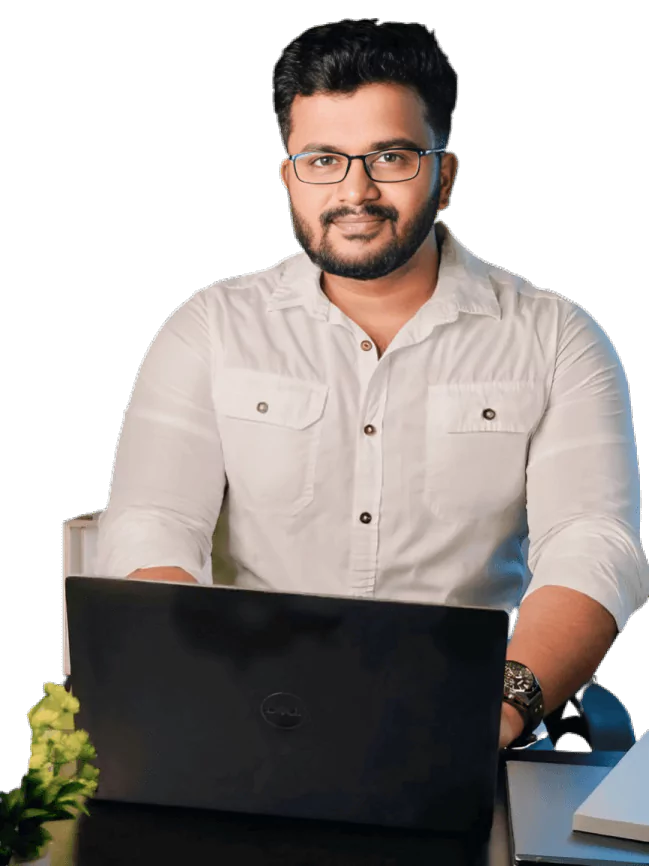
Ajesh Rana
Published on 02-08-2024
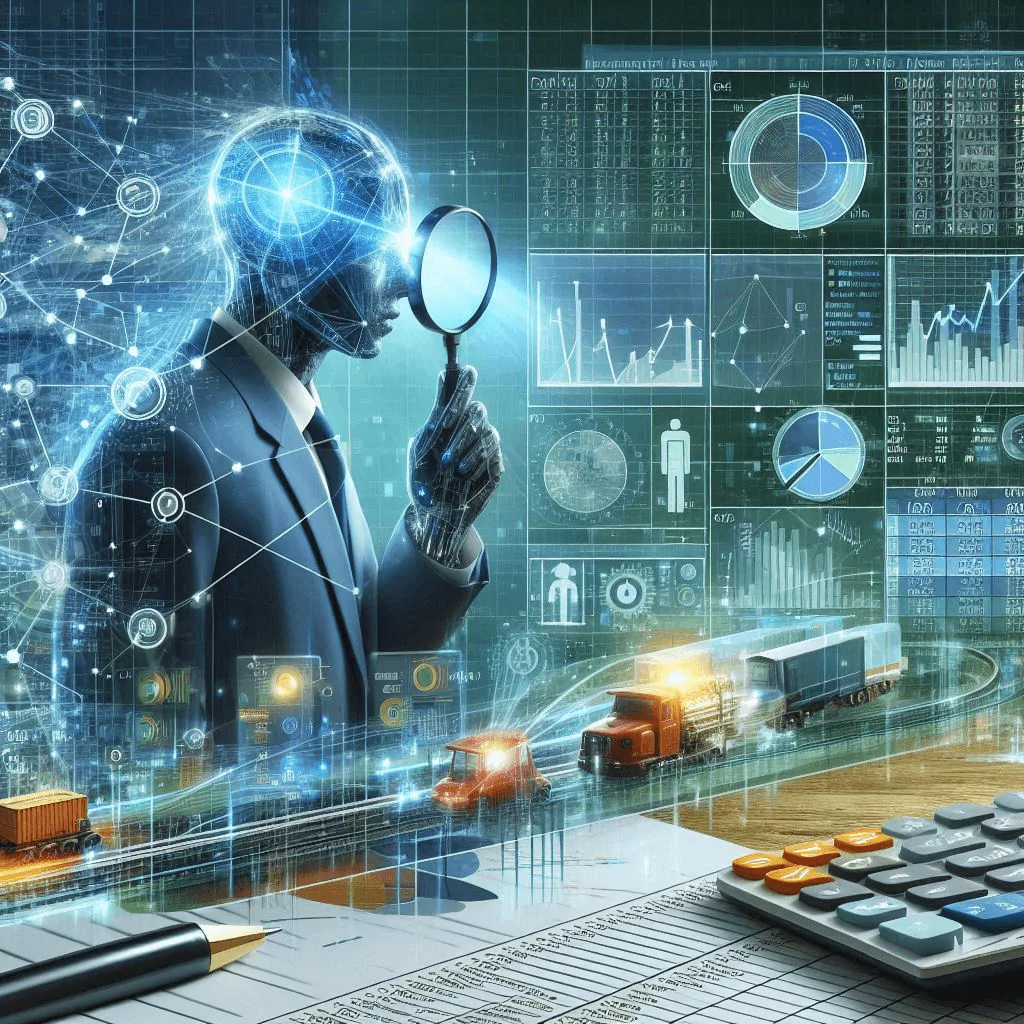
Introduction
It is the basic step in any data science project: Data Collection. The quality and nature of how you collect data can affect your analysis, affecting the performance levels. In this post, we will discuss the most common types of data collection method for both format and databases and code examples in Python to help you collect practical.
1. Collecting Data from CSV Files
CSV (Comma-Separated Values) files are a common format for storing tabular data. Pythons Pandas library provides an easy way to read from and write to CSV files.
import pandas as pd
# Reading data from a CSV file
df = pd.read_csv('data.csv')
print(df.head())
# Writing data to a CSV file
df.to_csv('output.csv', index=False)
Explanation:
pd.read_csv('data.csv')
: Reads data from a CSV file into a DataFrame.df.to_csv('output.csv', index=False)
: Writes data from a DataFrame to a CSV file without including row indices.
2. Collecting Data from Excel Files
Excel files are widely used for data storage and analysis. Pandas supports reading from and writing to Excel files using read_excel
and to_excel
.
import pandas as pd
# Reading data from an Excel file
df = pd.read_excel('data.xlsx', sheet_name='Sheet1')
print(df.head())
# Writing data to an Excel file
df.to_excel('output.xlsx', sheet_name='Sheet1', index=False)
Explanation:
pd.read_excel('data.xlsx', sheet_name='Sheet1')
: Reads data from a specified sheet in an Excel file.df.to_excel('output.xlsx', sheet_name='Sheet1', index=False)
: Writes data to a specified sheet in an Excel file without including row indices.
3. Collecting Data from HTML Tables
HTML tables can be parsed using Pandas. This is useful for the extracting data from web pages.
import pandas as pd
# Reading data from an HTML table
df = pd.read_html('https://example.com/table')[0]
print(df.head())
Explanation:
pd.read_html('https://example.com/table')[0]
: Reads the first table from the specified URL into a DataFrame.
4. Collecting Data from JSON Files
JSON (JavaScript Object Notation) is a flexible format for storing data. Pandas can handle JSON data easily.
import pandas as pd
# Reading data from a JSON file
df = pd.read_json('data.json')
print(df.head())
# Writing data to a JSON file
df.to_json('output.json', orient='records')
Explanation:
pd.read_json('data.json')
: Reads data from a JSON file into a DataFrame.df.to_json('output.json', orient='records')
: Writes data from a DataFrame to a JSON file in a record-oriented format.
5. Collecting Data from SQL Databases
Pandas can interact with SQL databases using pandas.io.sql
. Hereβs how to work with MySQL and PostgreSQL.
MySQL Example:
import pandas as pd
import pandas.io.sql as sqlio
import mysql.connector
# Connect to MySQL database
conn = mysql.connector.connect(
host='localhost',
user='username',
password='password',
database='database_name'
)
# Reading data from a SQL query
df = sqlio.read_sql_query('SELECT * FROM table_name', conn)
print(df.head())
# Writing data to a SQL table
df.to_sql('table_name', conn, if_exists='replace', index=False)
PostgreSQL Example:
import pandas as pd
import pandas.io.sql as sqlio
import psycopg2 as ps
# Connect to PostgreSQL database
conn = ps.connect(
dbname='database_name',
user='username',
password='password',
host='localhost'
)
# Reading data from a SQL query
df = sqlio.read_sql_query('SELECT * FROM table_name', conn)
print(df.head())
# Writing data to a SQL table
df.to_sql('table_name', conn, if_exists='replace', index=False)
Explanation:
sqlio.read_sql_query('SELECT * FROM table_name', conn)
: Executes a SQL query and loads the results into a DataFrame.df.to_sql('table_name', conn, if_exists='replace', index=False)
: Writes data to a SQL table, replacing it if it exists.
6. Collecting Data from MongoDB
MongoDB is a most popular NoSQL database. Use PyMongo to interact with MongoDB.
import pymongo
from pymongo import MongoClient
# Connect to MongoDB
client = MongoClient('mongodb://localhost:27017/')
db = client['database_name']
collection = db['collection_name']
# Reading data from MongoDB
data = collection.find()
df = pd.DataFrame(list(data))
print(df.head())
# Writing data to MongoDB
df.to_dict('records')
collection.insert_many(df.to_dict('records'))
Explanation:
collection.find()
: Retrieves documents from a MongoDB collection.pd.DataFrame(list(data))
: Converts the retrieved data into a DataFrame.df.to_dict('records')
: Converts DataFrame to a list of dictionaries for insertion into MongoDB.
7. Collecting Data from S3 (Amazon Simple Storage Service)
S3 is a cloud storage service provided by Amazon. You can use both boto3
and s3fs
to interact with S3 for data retrieval, visualization, and uploading.
# Import necessary libraries
import boto3
import pandas as pd
import s3fs
import matplotlib.pyplot as plt
# Initialize boto3 client and resource
s3_client = boto3.client(
service_name='s3',
region_name='us-east-2',
aws_access_key_id='your_access_key_id', # Replace with your AWS access key ID
aws_secret_access_key='your_secret_access_key' # Replace with your AWS secret access key
)
s3_resource = boto3.resource(
service_name='s3',
region_name='us-east-2',
aws_access_key_id='your_access_key_id', # Replace with your AWS access key ID
aws_secret_access_key='your_secret_access_key' # Replace with your AWS secret access key
)
# Initialize s3fs
fs = s3fs.S3FileSystem(
region='us-east-2',
key='your_access_key_id', # Replace with your AWS access key ID
secret='your_secret_access_key' # Replace with your AWS secret access key
)
# Function to list objects in a specific bucket using boto3
def list_objects_boto3(bucket_name):
print(f"Objects in bucket '{bucket_name}':")
for obj in s3_resource.Bucket(bucket_name).objects.all():
print(obj.key)
# Function to download a file from S3 using boto3
def download_file_boto3(bucket_name, object_key, local_file_path):
s3_client.download_file(bucket_name, object_key, local_file_path)
print(f"Downloaded {object_key} from bucket {bucket_name} to {local_file_path}")
# Function to read a CSV file from S3 into a DataFrame using s3fs
def read_csv_from_s3(bucket_name, object_key):
s3_path = f'{bucket_name}/{object_key}'
df = pd.read_csv(f's3://{s3_path}', storage_options={'s3fs': fs})
return df
# Function to visualize data using matplotlib
def visualize_data(df):
plt.figure(figsize=(10, 6))
df.plot(kind='line', x='Date', y='Value', title='Sample Data Visualization')
plt.xlabel('Date')
plt.ylabel('Value')
plt.grid(True)
plt.show()
# Function to upload a DataFrame to S3 using s3fs
def upload_dataframe_to_s3(df, bucket_name, object_key):
s3_path = f'{bucket_name}/{object_key}'
with fs.open(s3_path, 'w') as f:
df.to_csv(f, index=False)
print(f"Uploaded DataFrame to {s3_path}")
# Example usage
bucket_name = 'your_bucket_name' # Replace with your bucket name
object_key = 'path/to/your/file.csv' # Replace with the S3 object key
local_file_path = 'local/path/to/save/file.csv' # Replace with your local file path
# List objects in a bucket
list_objects_boto3(bucket_name)
# Download a file from S3
download_file_boto3(bucket_name, object_key, local_file_path)
# Read a CSV file from S3 into a DataFrame
df = read_csv_from_s3(bucket_name, object_key)
print(df.head())
# Visualize the data
visualize_data(df)
# Upload a DataFrame to S3
upload_dataframe_to_s3(df, bucket_name, 'path/to/uploaded/file.csv')
Explanation:
boto3.client()
andboto3.resource()
: Initializes the S3 client and resource to interact with S3.s3fs.S3FileSystem()
: Initializes the S3 file system interface for reading from and writing to S3.list_objects_boto3(bucket_name)
: Lists all objects in the specified S3 bucket.download_file_boto3(bucket_name, object_key, local_file_path)
: Downloads a file from S3 to a local path.read_csv_from_s3(bucket_name, object_key)
: Reads a CSV file from S3 into a pandas DataFrame usings3fs
.visualize_data(df)
: Usesmatplotlib
to visualize data from the DataFrame.upload_dataframe_to_s3(df, bucket_name, object_key)
: Uploads a DataFrame to S3 as a CSV file usings3fs
.
8. Collecting Data from a Public API
You can retrieve data from any public API URL using Python. Below is an example of how to fetch data from a public API and process it using the requests library.
import requests
import pandas as pd
# URL of the public API
api_url = 'https://api.example.com/data'
# Fetching data from the API
response = requests.get(api_url)
data = response.json()
# Converting data to a DataFrame
df = pd.DataFrame(data)
# Display the first few rows of the DataFrame
print(df.head())
# Example of saving the DataFrame to a CSV file
df.to_csv('output.csv', index=False)
Explanation:
requests.get(api_url)
: Fetches data from the public API URL.response.json()
: Parses the response as JSON.pd.DataFrame(data)
: Converts the JSON data into a DataFrame.df.to_csv('output.csv', index=False)
: Saves the DataFrame to a CSV file.
Conclusion
Data collection's is critical part of a data science,Β and understanding how to handle various formats and databases efficiently can enhance your workflow. By mastering these methods are you can ensure that your data is collected accurately and is ready for analysis.