The Data Analyst Guide to Best Practices for Data Analysis in Machine Learning Projects
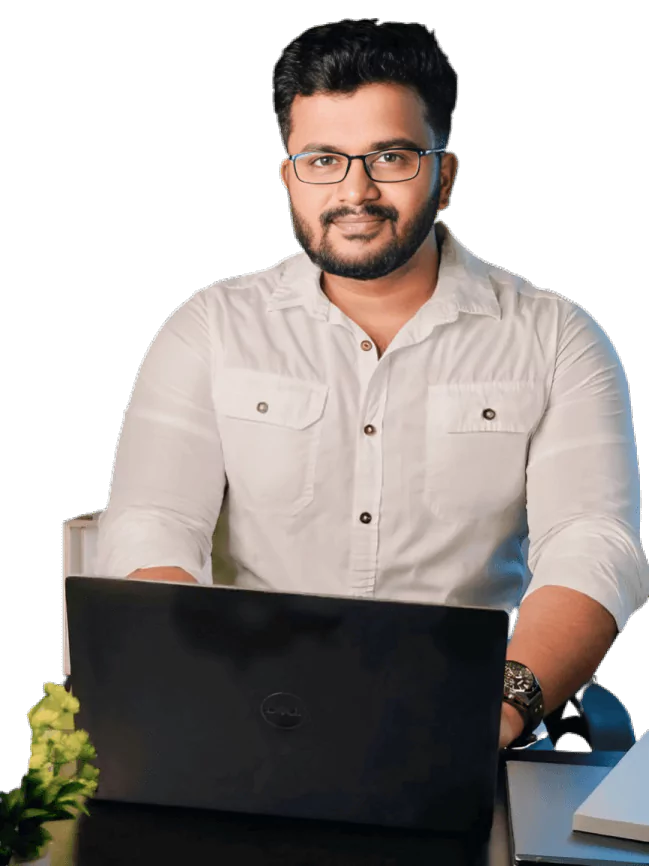
Ajesh Rana
Published on 02-08-2024
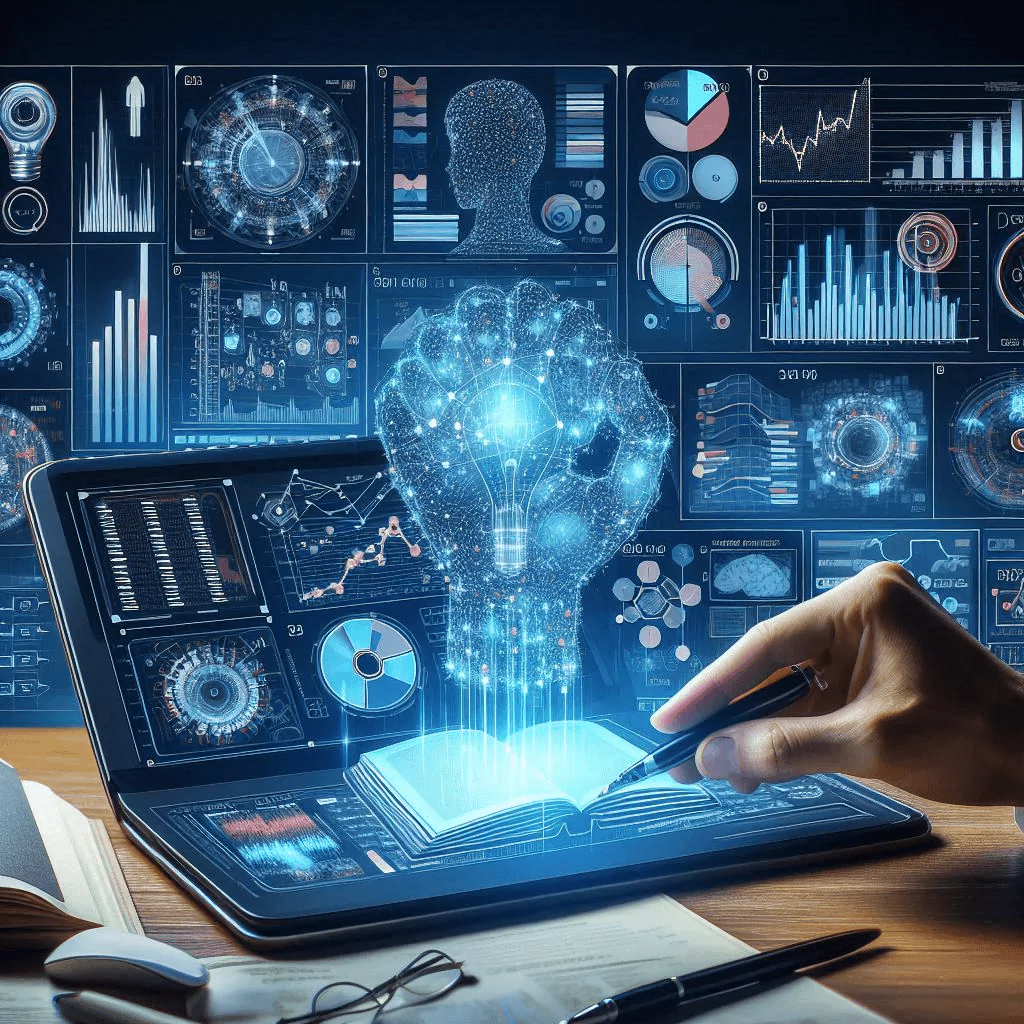
Introduction
Having good data is the fundamental need in a Data Science. as it makes analysis accurate and results more insightful. A data analyst is crucially involved in this phase and he/she helps meticulously gather, as well as analyse the necessary data. In short, this guide will give a clear blueprint to interpret data and intuit patterns/relationships in any ML project starting from scratch. Both of these step's are highly important in order to be preventative and get meaningful data from your work. Today we are discussing aspects of combining datasets, analysis on individual datasets and followed by practical implementation with python code along visualization output.
1. Combining Multiple Datasets
Why Combine Datasets?: The need to integrate data from multiple sources for a comprehensive analysis.
Techniques for Combining Datasets:
- Merge: Combining datasets based on common columns (keys).
- Concat: Stacking datasets either vertically or horizontally.
import pandas as pd
# Load datasets
data1 = pd.read_csv('data1.csv')
data2 = pd.read_csv('data2.csv')
# Merging datasets
merged_data = pd.merge(data1, data2, on='common_column')
# Concatenating datasets
concatenated_data = pd.concat([data1, data2], axis=0)
# Display the first few rows of the merged dataset
print(merged_data.head())
2. Receiving the Data
Understanding the Source: Importance of knowing the data source.
Initial Data Inspection: Overview of the dataset (rows, columns, types).
# Load the data
data = pd.read_csv('data.csv')
# Initial inspection
print(data.info())
print(data.head())
# Additional Steps:
# Check Dataset Shape:
print(data.shape)
# Check for Duplicate Columns:
print(data.columns.duplicated().sum())
# Display All Column Names:
print(data.columns)
3. Understanding Data Quality
Why Data Quality Matters: Impact of poor data quality on analysis.
Data Quality Dimensions: Accuracy, completeness, consistency, and validity.
4. Checking Missing Values
Why It's Important: Missing data can skew analysis.
Techniques for Handling Missing Data:
- Imputation
- Removal
# Checking for missing values
missing_values = data.isnull().sum()
missing_values = data.isna().sum()
print(missing_values)
# Handling missing values (example: filling with mean)
data.fillna(data.mean(), inplace=True)
data.fillna(data.median(), inplace=True)
data.fillna(data.mode[0](), inplace=True)
5. Identifying Duplicates
Impact of Duplicates: Distortion of analysis due to duplicates.
Detection and Removal: Techniques for identifying and removing duplicates.
# Checking for duplicates
duplicates = data.duplicated().sum()
print(f"Number of duplicates: {duplicates}")
# Removing duplicates
data = data.drop_duplicates()
6. Data Type Check
Why Data Types Matter: Correct data types ensure accurate analysis.
Conversion Techniques: Converting data types where necessary.
# Checking data types
print(data.dtypes)
# Converting data types
data['column_name'] = data['column_name'].astype('int')
data['column_name'] = data['column_name'].astype('str')
7. Error and Noisy Data Detection
Common Errors: Typos, out-of-range values, inconsistent formats.
Handling Noisy Data: Techniques to clean and preprocess noisy data.
# Detecting errors
invalid_data = data[data['column_name'] > threshold_value]
print(invalid_data)
# Cleaning noisy data
data['column_name'] = data['column_name'].apply(lambda x: clean_function(x))
8. Visualizing the Data
Importance of Visualization: Helps in understanding data distributions and patterns.
Common Visualization Techniques: Histograms, scatter plots, and box plots.
Why EDA is Crucial: Discovering patterns and trends.
Key EDA Techniques: Univariate, bivariate, and multivariate analysis.
import seaborn as sns
import matplotlib.pyplot as plt
# Histogram
plt.hist(data['column_name'])
plt.title('Distribution of Column Name')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.show()
# Scatter Plot
plt.scatter(data['x_column'], data['y_column'])
plt.title('Scatter Plot of X vs Y')
plt.xlabel('X')
plt.ylabel('Y')
plt.show()
# Univariate analysis
sns.histplot(data['column_name'], kde=True)
plt.show()
# Bivariate analysis
sns.scatterplot(x='column1', y='column2', data=data)
plt.show()
9. Hypothetical Analysis
Testing Hypotheses: Conducting hypothetical tests.
Why It's Important: Understanding underlying assumptions.
from scipy.stats import ttest_ind
# Hypothetical analysis example
group1 = data[data['group'] == 'A']['value']
group2 = data[data['group'] == 'B']['value']
t_stat, p_value = ttest_ind(group1, group2)
print(f"T-statistic: {t_stat}, P-value: {p_value}")
10. Data Correlation Check
Why Correlation Matters: Understanding relationships between variables.
Correlation Matrix: Visualizing correlations.
# Correlation matrix
correlation_matrix = data.corr()
sns.heatmap(correlation_matrix, annot=True, cmap='coolwarm')
plt.show()
11. Value Counts Check
Importance of Frequency Distribution: Analyzing the frequency of categorical values.
How to Use Value Counts: Detect anomalies and understand distribution.
# Value counts
value_counts = data['column_name'].value_counts()
print(value_counts)
12. Outlier Detection
Why Detect Outliers: Identifying data points that can skew analysis.
Techniques for Detecting Outliers: Z-score, IQR, and visual methods.
# Detecting outliers using Z-score
from scipy.stats import zscore
data['z_score'] = zscore(data['column_name'])
outliers = data[data['z_score'].abs() > 3]
print(outliers)
# Visual method (Boxplot)
sns.boxplot(x=data['column_name'])
plt.show()
Conclusion
So in the end, data analysis is an essential skill for any work done by a Data Science person. In this guide we covered the key steps that are required to prepare and analyze data. With these are strategies and Python code implemented, you will enhance your data analysis skills which are essential for anyone who wants to deliver significant insights in the field of Data Science.
We strongly recommend you to use the accompanying Jupyter Notebook that has all of this code readily available with explanation for a super easy integration.